Custom Game Engine
Along with our capstone studio responsibilities, programmers at FIEA are tasked with creating our own custom game engine from scratch written in C++.
This has been the most important project during my training, as its goal is to not only teach us C++ skills and best practices, but also to gain a complete understanding of how modern day game engines function at a base level.
Through the creation of this engine I have:
Gained strong knowledge of C++ syntax and the expressive potential of the language
Learned to grow and maintain a large code base that continues to build off itself
Developed a unit testing framework and debugging process that can be used throughout my career
Learned to use Visual Studios to create, maintain, and distribute projects in an industry standard fashion
Used JSON to master the deserialization process necessary for most applications
Gained a complete understanding of game engine structure and the process of exposing raw data to a scripting engine.
​
Below you will find examples of engine functionality that highlight the unique experience that this project has given me.
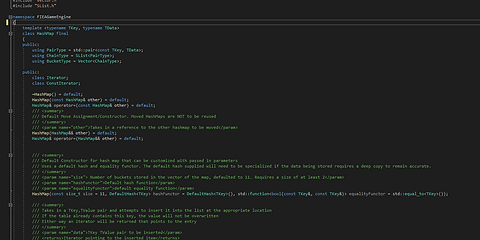
Custom Library Containers
Implemented my own Linked List, Vector, and Hashmap to gain a better understanding low level data structures
Gained a deep understanding of C++ Move/Copy Semantics
Practiced dynamic programming using C++Templates
Implemented custom Iterators to work with STL container interface
Hierarchical Scripting Language
Created a system that allows native C++ game data to be stored and exposed to the custom JSON language
​
Datum - structure that allows internal and external storage of data as primitives, user defined types, or Scopes
Scope - recursive relationship with Datum is used to form a hierarchical database
Attributed - binds data to the engine by mirroring native C++ classes as Datum/Scope objects that can be manipulated through JSON
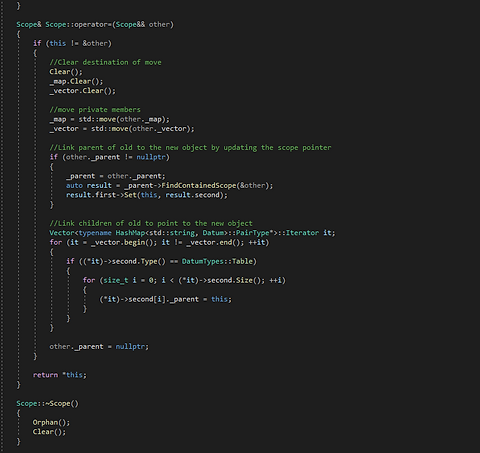
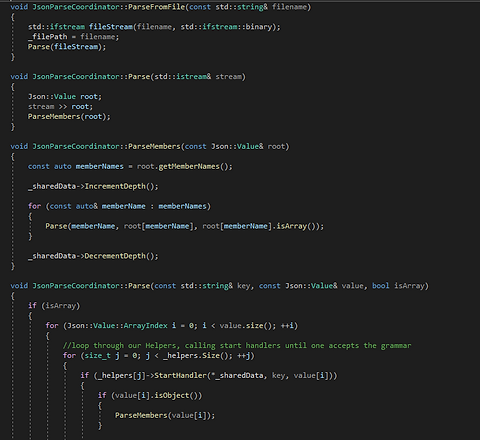
JSON Parsing System
Wrote a system to deserialize data at runtime, allowing for JSON to act as the scripting language for the engine
​
Used the chain of responsibility pattern to allow an extendable coordinator/helper system
Custom JSON grammar used to structure game object hierarchy
Practice working with abstract base classes/inheritance
Game Object
Designed an entity system similar to modern game engines that defines a base structure for objects that will make up the game world
​
Signature system uses Datum/Scope/Attributed to express common attributes (Transform, Name, Parent/Child Structure)
Type Manager allows custom types with the extension of game objects through C++ Inheritance
Update loop implemented through the Scope hierarchy
Factory pattern used to allow construction of user defined types at runtime
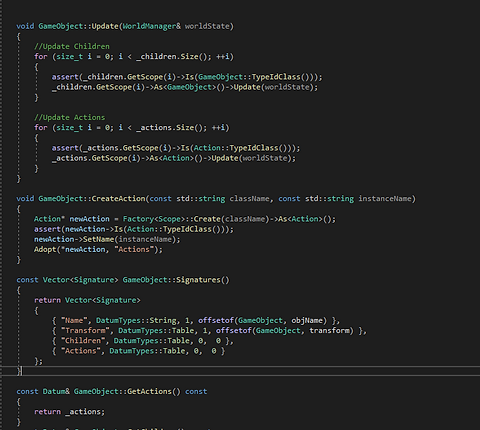
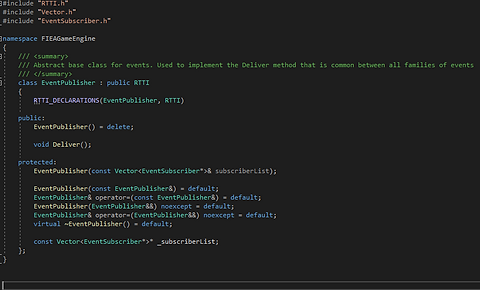
Action & Event
Developed a system that allows game objects to exhibit behaviors through the encapsulation of functionality within the Scope hierarchy
​
Action - extendable interface that allows user defined C++ logic to be nested within the GameObject hierarchy, adhering to the update loop based on adjustable conditions
​
Event - templated queue system that implements the observer pattern to allow GameObjects to subscribe and react to the behaviors of other GameObjects
Unit Testing Framework
Structured a project testing environment using Visual Studio's CPPUnitTestFramework
Maintained testing methods throughout project lifecycle, developing modules for each individual class
Wrote extensive unit tests to exhaust edge cases and discover bugs before continuing development


Super PoggerMan
At the end of the project, teams were formed to create a game using the engine. We created a final version based on the work we all had done, and made a Bomberman clone that combined our engines functionality along with SDLs rendering framework
​
​BomberMan gameplay emulated through a dynamic tile system
Use of custom engine functionality seamlessly combined with SDL framework
Fully customizable game maps through JSON